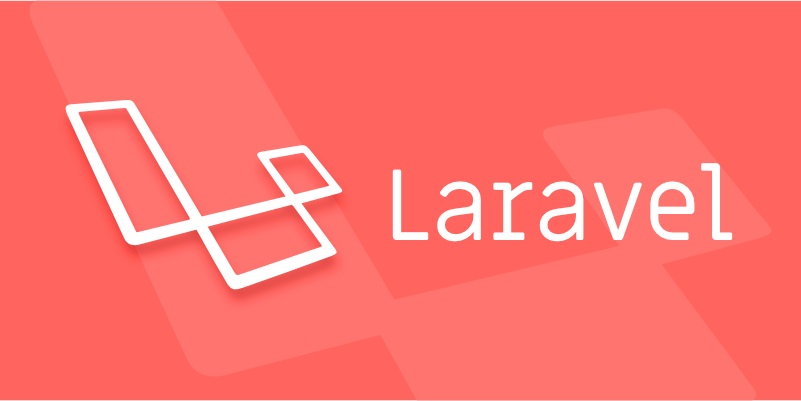
Database seeder is used for generating fake data for testing purpose. It helps to reduce the developer’s time by avoiding data insertion in the database table.
Step-1: Create Model named Employee and Run Migration
php artisan make:model Employee -m
Step-2: Now go to the migtation table file (database/migrations/timestamp_employees_table.php) for defining table fields.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateEmployeesTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('employees', function (Blueprint $table) {
$table->id();
$table->String('name');
$table->String('email');
$table->String('phone');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('employees');
}
}
Step-3: Similarly, define the Employee table in app/Models/Employee.php:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Employee extends Model
{
use HasFactory;
protected $table = "employees";
}
Step-4: Now run migrate command
php artisan migrate
Step-5: now go the DatabaseSeeder.php file (Database\seeders\ DatabaseSeeder.php)
<?php
namespace Database\Seeders;
use Illuminate\Database\Seeder;
use Illuminate\Support\Facades\DB;
use Faker\Factory as Faker;
class DatabaseSeeder extends Seeder
{
/**
* Seed the application's database.
*
* @return void
*/
public function run()
{
// \App\Models\User::factory(10)->create();
$faker = Faker::create();
foreach(range(1,100) as $index){
DB::table('employees')->insert([
'name' => $faker->name,
'email' => $faker->email,
'phone' => $faker->phoneNumber
]);
}
}
}
Step-6: Now run the below code and you will see in emplyee table that 100 datas have been inserted automatically!
composer dump-autoload
php artisan db:seed
[fb_button]