In this project I will show you how to add two numbers and show result. For that I will take Two input fields and a submit button. However, I will create a separate dart file to keep my all style of widgets like text field, button.
Here I will create two dart files under lib directory which are main.dart and styIe.dart.
In main.dart file write the below code:
import 'package:flutter/material.dart'; import 'package:flutter_sum_app/style.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( title: "Summation App", home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { Map<String, double> FormValue = {"num1": 0, "num2": 0}; double SUM = 0; @override Widget build(BuildContext context) { MyInputOnChange(InputKey, InputValue) { setState(() { FormValue.update(InputKey, (value) => double.parse(InputValue)); }); } AddAllNumber() { setState(() { SUM = FormValue["num1"]! + FormValue["num2"]!; }); } return Scaffold( appBar: AppBar( title: Text("Sum App"), ), body: Padding( padding: EdgeInsets.all(40), child: Column( mainAxisAlignment: MainAxisAlignment.start, children: [ Text( SUM.toString(), style: HeadTextStyle(), ), SizedBox( height: 20, ), TextFormField( onChanged: (value) { // set value to num1 MyInputOnChange("num1", value); }, decoration: AppInputStyle("First Number"), ), SizedBox( height: 20, ), TextFormField( onChanged: (value) { MyInputOnChange("num2", value); }, decoration: AppInputStyle("Last Number"), ), SizedBox( height: 20, ), Container( width: double.infinity, child: ElevatedButton( onPressed: () { AddAllNumber(); }, style: AppButtonStyle(), child: Text("Add"))) ], ), ), ); } }
Now write the below code in style.dart file
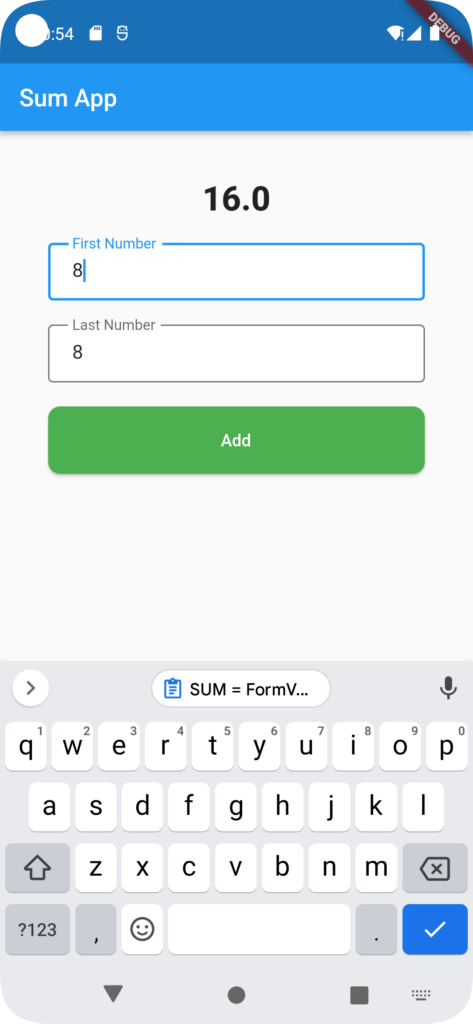
import 'package:flutter/material.dart'; InputDecoration AppInputStyle(label){ return InputDecoration( contentPadding: EdgeInsets.fromLTRB(20, 10, 10, 10), filled: true, fillColor: Colors.white, border: OutlineInputBorder(), labelText: label ); } TextStyle HeadTextStyle(){ return TextStyle( fontSize: 28, fontWeight: FontWeight.w800, ); } ButtonStyle AppButtonStyle(){ return ElevatedButton.styleFrom( padding: EdgeInsets.all(20), backgroundColor: Colors.green, shape: RoundedRectangleBorder( borderRadius: BorderRadius.all(Radius.circular(10)) ) ); }
Now run these files in Android Studio and you can now add numbers! However, if you want to learn this program using controller and with best practice follow the below link.