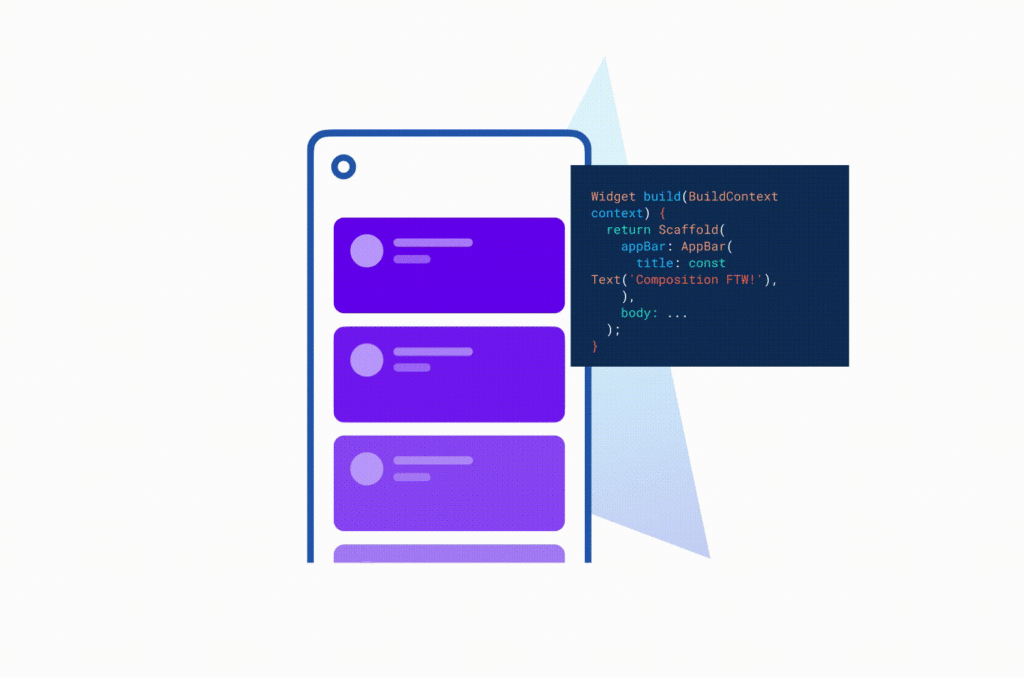
Table of Contents
Flutter
Stack and Positioned widget:
Creating Bangladesh Flag using Stack and Positioned widget in flutter!
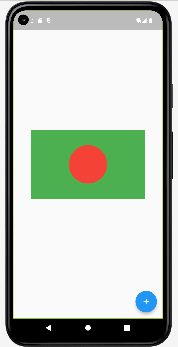
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, home: Scaffold( floatingActionButton: FloatingActionButton( onPressed: () {}, child: Icon(Icons.add), ), body: Center( child: Stack( alignment: Alignment.center, children: [ Container( height: 180, width: 300, color: Colors.green, ), Positioned( child: CircleAvatar( radius: 50, backgroundColor: Colors.red, ), ), ], ), ), ), ); } }
Responsive UI using Expanded Widget.
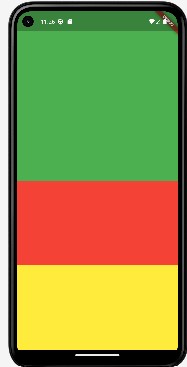
import 'package:flutter/material.dart'; import 'package:liquid_swipe/liquid_swipe.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( body: Column( children: [ Expanded( flex: 2, child: Container( color: Colors.green, )), Expanded( flex: 1, child: Container( color: Colors.red, )), Expanded( flex: 1, child: Container( color: Colors.yellow, )) ], ), ), ); } }
Hero Widget:
Here I will create a new file “second.dart” besides main.dart
// file name "main.dart" import 'package:dart_tutorial/second.dart'; import 'package:flutter/material.dart'; void main()=>runApp(MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp(home: Home(),); } } class Home extends StatelessWidget { const Home({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( body: Center( child: CircleAvatar( radius: 50, child: GestureDetector( child: Hero( tag: "add", child: Icon( Icons.add_a_photo, size: 50, ), ), onTap: (){ Navigator.push(context, MaterialPageRoute(builder: (context)=>second())); }, ), ), ), ); } }
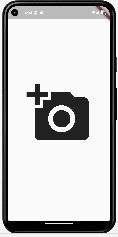
//file name "second.dart" import 'package:dart_tutorial/second.dart'; import 'package:flutter/material.dart'; void main()=>runApp(MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp(home: Home(),); } } class Home extends StatelessWidget { const Home({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( body: Center( child: CircleAvatar( radius: 50, child: GestureDetector( child: Hero( tag: "add", child: Icon( Icons.add_a_photo, size: 50, ), ), onTap: (){ Navigator.push(context, MaterialPageRoute(builder: (context)=>second())); }, ), ), ), ); } }
Actions in AppBar & Floating Action Button
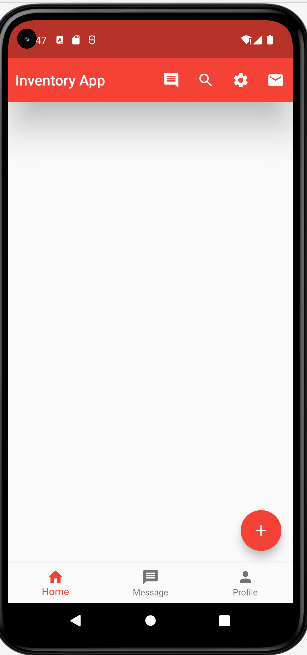
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( theme: ThemeData(primarySwatch: Colors.red), darkTheme: ThemeData(primarySwatch: Colors.amber), color: Colors.blue, debugShowCheckedModeBanner: false, home: HomeActivity(), ); } } class HomeActivity extends StatelessWidget { const HomeActivity({super.key}); MySnackBar(message, context) { return ScaffoldMessenger.of(context) .showSnackBar(SnackBar(content: Text(message))); } @override Widget build(BuildContext context) { return Scaffold( //Appbar section appBar: AppBar( title: Text("Inventory App"), titleSpacing: 10, toolbarHeight: 60, toolbarOpacity: 1, elevation: 40, actions: [ IconButton( onPressed: () { MySnackBar("Comments", context); }, icon: Icon(Icons.comment)), IconButton( onPressed: () { MySnackBar("Search", context); }, icon: Icon(Icons.search)), IconButton( onPressed: () { MySnackBar("Settings", context); }, icon: Icon(Icons.settings)), IconButton( onPressed: () { MySnackBar("Email", context); }, icon: Icon(Icons.email)) ], ), //Floating actionbutton section floatingActionButton: FloatingActionButton( elevation: 10, child: Icon(Icons.add), onPressed: () { MySnackBar("I am floating action button", context); }, ), //Bottom navigation bottomNavigationBar: BottomNavigationBar( currentIndex: 0, items: [ BottomNavigationBarItem(icon: Icon(Icons.home), label: "Home"), BottomNavigationBarItem(icon: Icon(Icons.message), label: "Message"), BottomNavigationBarItem(icon: Icon(Icons.person), label: "Profile"), ], onTap: (int index) { if (index == 0) { MySnackBar("I am Home", context); } if (index == 1) { MySnackBar("I am Message", context); } if (index == 2) { MySnackBar("I am Profile", context); } }, ), ); } }
Elevated, Text and Outline Button
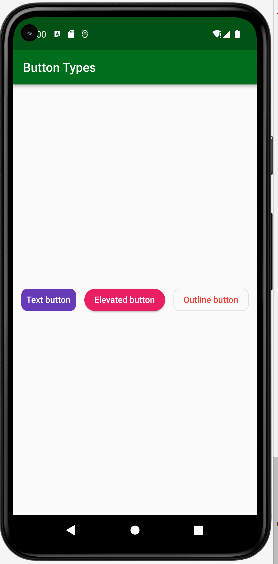
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.green), //useMaterial3: true, ), home: HomePage(), ); } } class HomePage extends StatelessWidget { const HomePage({Key? key}) : super(key: key); MySnackBar(message, context) { return ScaffoldMessenger.of(context) .showSnackBar(SnackBar(content: Text(message))); } @override Widget build(BuildContext context) { ButtonStyle buttonStyle2 = TextButton.styleFrom( backgroundColor: Colors.deepPurple, foregroundColor: Colors.white, shape: RoundedRectangleBorder( borderRadius: BorderRadius.all(Radius.circular(10)))); ButtonStyle buttonStyle = ElevatedButton.styleFrom( backgroundColor: Colors.pink, foregroundColor: Colors.white, shape: RoundedRectangleBorder( borderRadius: BorderRadius.all(Radius.circular(20)))); ButtonStyle buttonStyle1 = OutlinedButton.styleFrom( foregroundColor: Colors.red, shape: RoundedRectangleBorder( borderRadius: BorderRadius.all(Radius.circular(10)))); return Scaffold( appBar: AppBar( title: Text("Button Types"), ), body: Center( child: Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: [ TextButton( onPressed: () { MySnackBar("Text Button is pressed", context); }, child: Text('Text button'), style: buttonStyle2, ), ElevatedButton( onPressed: () { MySnackBar("Elevated Button is pressed", context); }, child: Text('Elevated button'), style: buttonStyle, ), OutlinedButton( onPressed: () { MySnackBar("Outline Button is pressed", context); }, child: Text('Outline button'), style: buttonStyle1,), ], ), ), ); } }
Circular Progress and Linear Progress
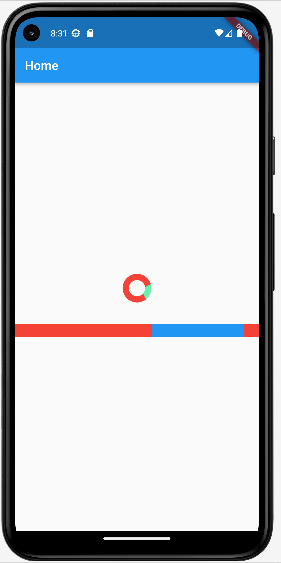
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( //colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple), useMaterial3: false, ), home: const MyHomePage(), ); } } class MyHomePage extends StatelessWidget { const MyHomePage({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Home"), ), body: const Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ CircularProgressIndicator( color: Colors.red, strokeWidth: 10, backgroundColor: Colors.greenAccent, ), SizedBox( height: 40, ), LinearProgressIndicator( color: Colors.blue, minHeight: 20, backgroundColor: Colors.red, ) ], ), ), ); } }
Card in Flutter
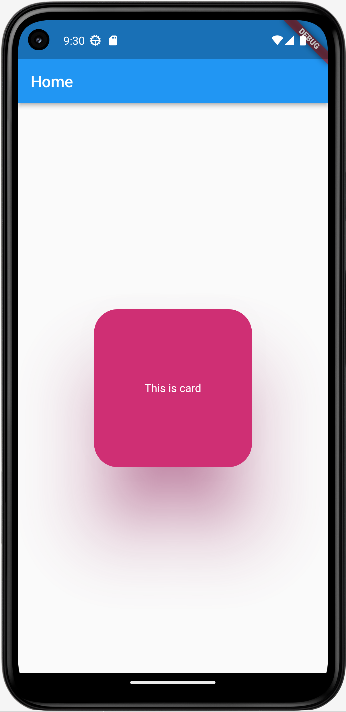
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( //colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple), useMaterial3: false, ), home: const MyHomePage(), ); } } class MyHomePage extends StatelessWidget { const MyHomePage({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text("Home"), ), body: Center( child: Card( shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(30)), shadowColor: const Color.fromRGBO(227, 61, 148, 1), color: const Color.fromRGBO(207, 47, 116, 1), elevation: 80, child: const SizedBox( height: 200, width: 200, child: Center( child: Text( 'This is card', style: TextStyle(color: Colors.white), ), ), ), ), ), ); } }
Navigator with parameter passing
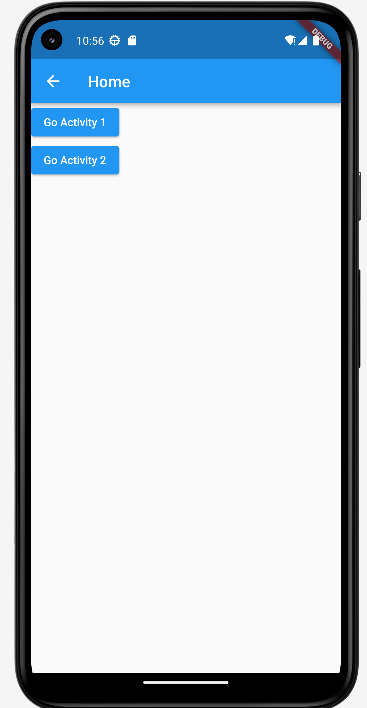
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( //colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple), useMaterial3: false, ), home: const MyHomePage(), ); } } class MyHomePage extends StatelessWidget { const MyHomePage({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text("Home"), ), body: Column( mainAxisAlignment: MainAxisAlignment.start, children: [ ElevatedButton( onPressed: () { Navigator.push( context, MaterialPageRoute( builder: (context) => PageActivity1("This is from activity1"))); }, child: Text("Go Activity 1")), ElevatedButton( onPressed: () { Navigator.push( context, MaterialPageRoute( builder: (context) => PageActivity2("This is from activity2"))); }, child: Text("Go Activity 2")), ], ), ); } } class PageActivity1 extends StatelessWidget { String msg; PageActivity1(this.msg, {Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(msg), ), body: Center( child: ElevatedButton( onPressed: () { Navigator.push( context, MaterialPageRoute( builder: (context) => PageActivity2("This is from activity2"))); }, child: Text("Go Activity 2")), ), ); } } class PageActivity2 extends StatelessWidget { String msg; PageActivity2(this.msg, {Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(msg), ), body: Center( child: ElevatedButton( onPressed: () { Navigator.push(context, MaterialPageRoute(builder: (context) => MyHomePage())); }, child: Text("Go Home")), ), ); } }
Alert Dialogue in Flutter
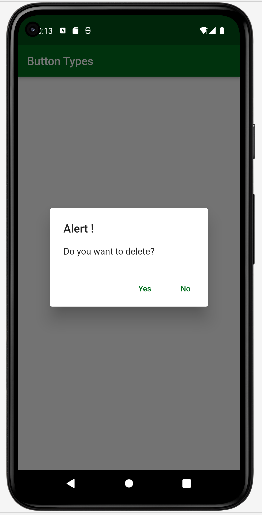
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.green), //useMaterial3: true, ), home: HomePage(), ); } } class HomePage extends StatelessWidget { const HomePage({Key? key}) : super(key: key); MySnackBar(message, context) { return ScaffoldMessenger.of(context) .showSnackBar(SnackBar(content: Text(message))); } MyAlertDialogue(context) { return showDialog( context: context, builder: (BuildContext context) { return Expanded( child: AlertDialog( title: Text("Alert !"), content: Text("Do you want to delete?"), actions: [ TextButton( onPressed: () { MySnackBar("Delete successfully", context); Navigator.of(context).pop(); }, child: Text("Yes")), TextButton( onPressed: () { Navigator.of(context).pop(); }, child: Text("No")) ], ), ); }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Button Types"), ), body: Center( child: Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: [ ElevatedButton( onPressed: () { MyAlertDialogue(context); }, child: Text('Elevated button')), ], ), ), ); } }
Simple Form with Text Field
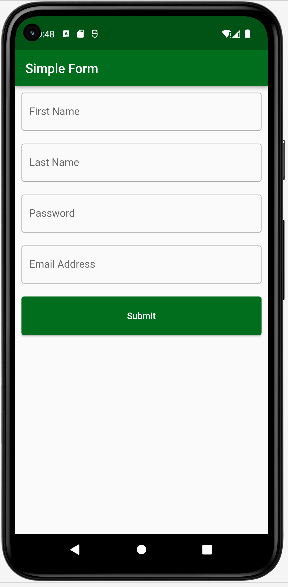
import 'package:flutter/material.dart'; void main() {import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.green), ), home: HomePage(), ); } } class HomePage extends StatelessWidget { const HomePage({Key? key}) : super(key: key); @override Widget build(BuildContext context) { ButtonStyle buttonStyle = ElevatedButton.styleFrom(minimumSize: Size(double.infinity, 60)); return Scaffold( appBar: AppBar( title: Text("Simple Form"), ), body: Column( mainAxisAlignment: MainAxisAlignment.start, children: [ Padding( padding: EdgeInsets.all(10), child: TextField( decoration: InputDecoration( border: OutlineInputBorder(), labelText: "First Name", hintText: 'Enter your First Name'), ), ), Padding( padding: EdgeInsets.all(10), child: TextField( decoration: InputDecoration( border: OutlineInputBorder(), labelText: "Last Name", hintText: 'Enter your Last Name'), ), ), Padding( padding: EdgeInsets.all(10), child: TextField( obscureText: true, decoration: InputDecoration( border: OutlineInputBorder(), labelText: "Password", hintText: 'Enter your password'), ), ), Padding( padding: EdgeInsets.all(10), child: TextField( decoration: InputDecoration( border: OutlineInputBorder(), labelText: "Email Address", hintText: 'Enter your Email Address'), ), ), Padding( padding: EdgeInsets.all(10), child: ElevatedButton( onPressed: () {}, child: Text("Submit"), style: buttonStyle, ), ) ], ), ); } } runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.green), ), home: HomePage(), ); } } class HomePage extends StatelessWidget { const HomePage({Key? key}) : super(key: key); @override Widget build(BuildContext context) { ButtonStyle buttonStyle = ElevatedButton.styleFrom(minimumSize: Size(double.infinity, 60)); return Scaffold( appBar: AppBar( title: Text("Simple Form"), ), body: Column( mainAxisAlignment: MainAxisAlignment.start, children: [ Padding( padding: EdgeInsets.all(10), child: TextField( decoration: InputDecoration( border: OutlineInputBorder(), labelText: "First Name"), ), ), Padding( padding: EdgeInsets.all(10), child: TextField( decoration: InputDecoration( border: OutlineInputBorder(), labelText: "Last Name"), ), ), Padding( padding: EdgeInsets.all(10), child: TextField( decoration: InputDecoration( border: OutlineInputBorder(), labelText: "Email Address"), ), ), Padding( padding: EdgeInsets.all(10), child: ElevatedButton( onPressed: () {}, child: Text("Submit"), style: buttonStyle, ), ) ], ), ); } }
Simple ListView in Flutter
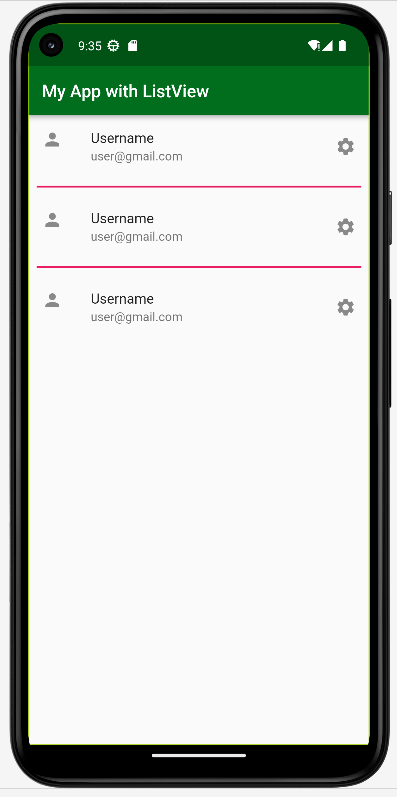
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.green), ), home: HomePage(), ); } } class HomePage extends StatelessWidget { HomePage({Key? key}) : super(key: key); MySnackBar(message, context) { return ScaffoldMessenger.of(context) .showSnackBar(SnackBar(content: Text(message))); } @override Widget build(BuildContext context) { ButtonStyle buttonStyle = ElevatedButton.styleFrom(minimumSize: Size(double.infinity, 60)); return Scaffold( appBar: AppBar( title: Text("My App with ListView"), ), body: ListView( children: [ ListTile( title: Text("Username"), subtitle: Text("user@gmail.com"), leading: Icon(Icons.person), trailing: Icon(Icons.settings), onTap: (){ print("Listtile pressed"); }, ), Divider(height: 20, thickness: 2, color: Colors.pink, indent: 10, endIndent: 10, ), ListTile( title: Text("Username"), subtitle: Text("user@gmail.com"), leading: Icon(Icons.person), trailing: Icon(Icons.settings), onTap: (){ print("Listtile pressed"); }, ), Divider(height: 20, thickness: 2, color: Colors.pink, indent: 10, endIndent: 10, ), ListTile( title: Text("Username"), subtitle: Text("user@gmail.com"), leading: Icon(Icons.person), trailing: Icon(Icons.settings), onTap: (){ print("Listtile pressed"); }, ), ], ), ); } }
Dynamic List View Builder With Gesture Detector
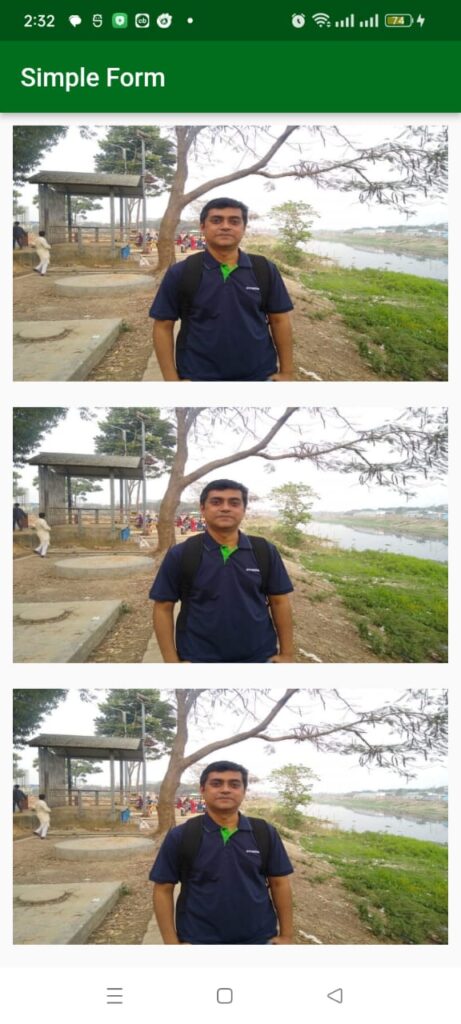
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.green), ), home: HomePage(), ); } } class HomePage extends StatelessWidget { HomePage({Key? key}) : super(key: key); MySnackBar(message, context) { return ScaffoldMessenger.of(context) .showSnackBar(SnackBar(content: Text(message))); } var myItems = [ { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Nazim" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Mamun" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Akif" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Zarif" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Sultana" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Sara" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Karim" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Niloy" }, ]; @override Widget build(BuildContext context) { ButtonStyle buttonStyle = ElevatedButton.styleFrom(minimumSize: Size(double.infinity, 60)); return Scaffold( appBar: AppBar( title: Text("My App with ListView and Scrollbar"), ), body: Scrollbar( thickness: 20, radius: Radius.circular(10), child: ListView.builder( itemCount: myItems.length, itemBuilder: (context, index) { return GestureDetector( onTap: () { MySnackBar(myItems[index]['title'], context); }, child: Container( margin: EdgeInsets.all(10), width: double.infinity, height: 200, child: Image.network( myItems[index]['img']!, fit: BoxFit.fill, ), ), ); }, ), ), ); } }
Simple GridView in Flutter
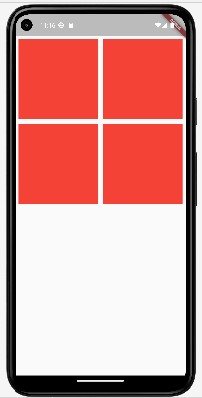
import 'package:flutter/material.dart'; import 'package:liquid_swipe/liquid_swipe.dart'; void main(){ runApp(MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( body: Padding( padding: const EdgeInsets.all(8.0), child: GridView.count(crossAxisCount: 2, mainAxisSpacing: 10, crossAxisSpacing: 10, children: [ Container( height: 200, width: 200, color: Colors.red, ), Container( height: 200, width: 200, color: Colors.red, ), Container( height: 200, width: 200, color: Colors.red, ), Container( height: 200, width: 200, color: Colors.red, ), ],), ) ), ); } }
Dynamic GridView in Flutter
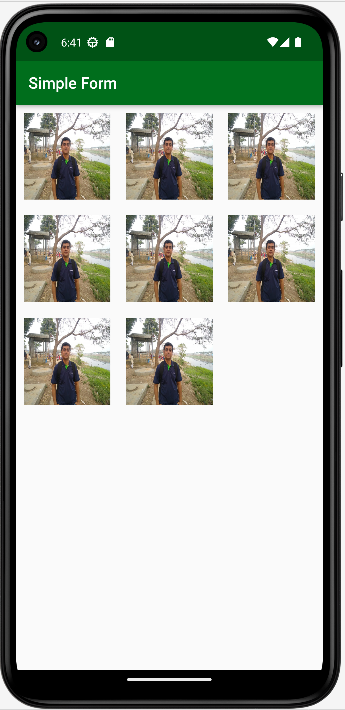
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.green), ), home: HomePage(), ); } } class HomePage extends StatelessWidget { HomePage({Key? key}) : super(key: key); MySnackBar(message, context) { return ScaffoldMessenger.of(context) .showSnackBar(SnackBar(content: Text(message))); } var myItems = [ { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Nazim" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Mamun" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Akif" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Zarif" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Sultana" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Sara" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Karim" }, { "img": "https://nazimuddin.xyz/wp-content/uploads/2021/04/Nazim-768x576.jpg", "title": "Niloy" }, ]; @override Widget build(BuildContext context) { ButtonStyle buttonStyle = ElevatedButton.styleFrom(minimumSize: Size(double.infinity, 60)); return Scaffold( appBar: AppBar( title: Text("Simple Form"), ), body: GridView.builder( gridDelegate: SliverGridDelegateWithFixedCrossAxisCount( // You can change the number of columns here crossAxisCount: 3, crossAxisSpacing: 1, childAspectRatio: 1.1 ), itemCount: myItems.length, itemBuilder: (context, index) { return GestureDetector( onTap: () { MySnackBar(myItems[index]['title'], context); }, child: Container( margin: EdgeInsets.all(5), width: double.infinity, height: 200, child: Image.network( myItems[index]['img']!, fit: BoxFit.fill, ), ), ); }, ), ); } }
GestureDetector and InkWell in Flutter
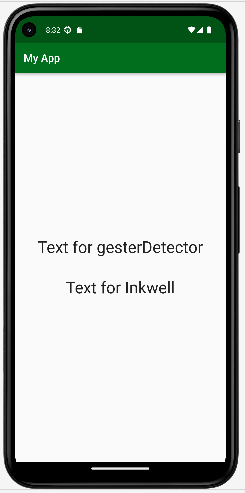
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.green), ), home: HomePage(), ); } } class HomePage extends StatelessWidget { HomePage({Key? key}) : super(key: key); import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.green), ), home: HomePage(), ); } } class HomePage extends StatelessWidget { HomePage({Key? key}) : super(key: key); @override Widget build(BuildContext context) { ButtonStyle buttonStyle = ElevatedButton.styleFrom(minimumSize: Size(double.infinity, 60)); return Scaffold( appBar: AppBar( title: Text("My App"), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ GestureDetector( onTap: () { print("Single pressed"); }, onDoubleTap: () { print("Double tapped"); }, onLongPress: () { print("Long tapped"); }, child: Text( "Text for gesterDetector", style: TextStyle(fontSize: 30), ), ), SizedBox( height: 40, ), InkWell( onTap: () { print("Single pressed"); }, onDoubleTap: () { print("Double tapped"); }, onLongPress: () { print("Long tapped"); }, child: Text( "Text for Inkwell", style: TextStyle(fontSize: 30), ), ) ], ), )); } } @override Widget build(BuildContext context) { ButtonStyle buttonStyle = ElevatedButton.styleFrom(minimumSize: Size(double.infinity, 60)); return Scaffold( appBar: AppBar( title: Text("My App"), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ GestureDetector( onTap: () { print("Single pressed"); }, onDoubleTap: () { print("Double tapped"); }, onLongPress: () { print("Long tapped"); }, child: Text( "Text for gesterDetector", style: TextStyle(fontSize: 30), ), ), SizedBox( height: 40, ), InkWell( onTap: () { print("Hello world"); }, onDoubleTap: () { print("Double tapped"); }, onLongPress: () { print("Long tapped"); }, child: Text( "Text for Inkwell", style: TextStyle(fontSize: 30), ), ) ], ), )); } }
Text Field, Grid, List and Floating Action Bar
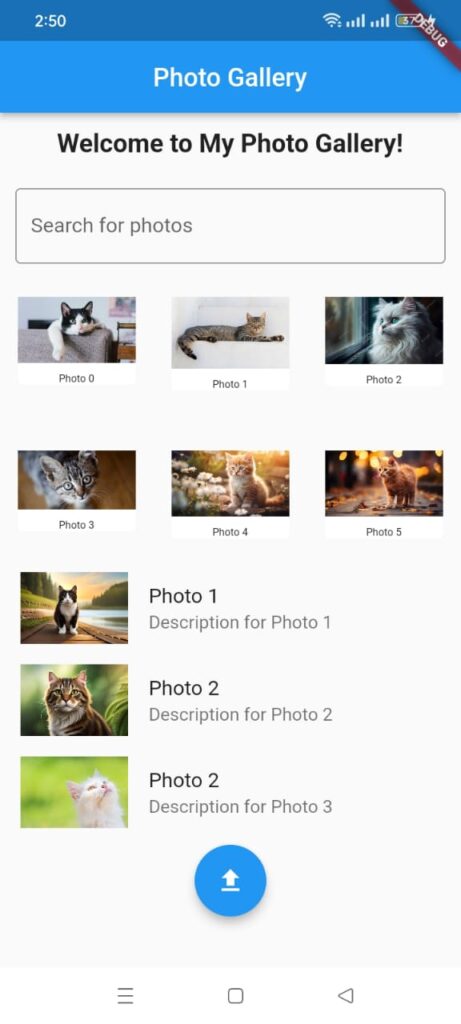
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( centerTitle: true, title: Text("Photo Gallery"), ), body: MyWidget(), ), ); } } class MyWidget extends StatefulWidget { @override _MyWidgetState createState() => _MyWidgetState(); } class _MyWidgetState extends State<MyWidget> { MySnackBar(message, context) { return ScaffoldMessenger.of(context) .showSnackBar(SnackBar(content: Text(message))); } var myItems = [ { "img": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTKboCkjXUKztIj7P8a5UjeFn0lAMQSp_TqhQ&usqp=CAU", "title": "Photo 0", "caption": "Caption 0", }, { "img": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcRr_-62a40u3lSIyRP5EKOjJeQiZROwTeVCOQ&usqp=CAU", "title": "Photo 1", "caption": "Caption 1", }, { "img": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcR0cf6jcfBR56-w0-_ChVozZfDmwh6F2z0NbQ&usqp=CAU", "title": "Photo 2", "caption": "Caption 2", }, { "img": "https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTnOrOwY43A2IXz1v0yLjmHVWj0d2_YMm_6eA&usqp=CAU", "title": "Photo 3", "caption": "Caption 3", }, { "img": "https://img.freepik.com/free-photo/beautiful-kitten-with-flowers-outdoors_23-2150752804.jpg?size=626&ext=jpg", "title": "Photo 4", "caption": "Caption 4", }, { "img": "https://img.freepik.com/free-photo/cute-kitten-walking-outdoors_23-2150752830.jpg?size=626&ext=jpg", "title": "Photo 5", "caption": "Caption 5", }, ]; var listItems = [ { "img": "https://img.freepik.com/premium-photo/cat-bridge-forest_865967-105980.jpg?size=626&ext=jpg", "title": "Photo 1", "caption": "Description for Photo 1", }, { "img": "https://img.freepik.com/premium-photo/cat-with-green-eyes-white-stripe-its-face_865967-105888.jpg?size=626&ext=jpg", "title": "Photo 2", "caption": "Description for Photo 2", }, { "img": "https://img.freepik.com/free-photo/white-cat-garden_1150-43925.jpg?size=626&ext=jpg&ga=GA1.2.1046328686.1694962208&semt=ais", "title": "Photo 2", "caption": "Description for Photo 3", }, ]; @override Widget build(BuildContext context) { return Column( children: <Widget>[ SingleChildScrollView( scrollDirection: Axis.horizontal, child: Padding( padding: EdgeInsets.all(12.0), child: Text( "Welcome to My Photo Gallery!", style: TextStyle( fontSize: 20.0, fontWeight: FontWeight.bold, ), ), ), ), Padding( padding: EdgeInsets.all(12.0), child: TextField( decoration: InputDecoration( labelText: "Search for photos", border: OutlineInputBorder(), ), ), ), Expanded( child: GridView.builder( gridDelegate: SliverGridDelegateWithFixedCrossAxisCount( crossAxisCount: 3, ), itemCount: myItems.length, itemBuilder: (context, index) { return GestureDetector( onTap: () { MySnackBar(myItems[index]['title'], context); }, child: Column( children: [ Card( elevation: 0.0, margin: EdgeInsets.all(14.0), child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Image.network( myItems[index]['img']!, fit: BoxFit.fill, ), SizedBox(height: 8.0), Text( myItems[index]['title']!, style: TextStyle( fontSize: 8.0, // fontWeight: FontWeight.bold, ), ), ], ), ), ], ), ); }, ), ), Expanded( child: ListView.builder( itemCount: listItems.length, itemBuilder: (context, index) { return ListTile( leading: Image.network( listItems[index]['img']!, fit: BoxFit.fill,), // You can customize the leading icon title: Text(listItems[index]['title']!), subtitle: Text(listItems[index]['caption']!), // Optional subtitle onTap: () { // Handle item tap here //print("Tapped on ${items[index]}"); }, ); }, ), ), Align( alignment: Alignment.center, child: FloatingActionButton( onPressed: () { MySnackBar("Photos Uploaded Successfully!", context); }, child: Icon(Icons.upload), ), ), SizedBox(height: 40.0), ], ); } }
Navigation, routing, data passing from one Activity to another Activity
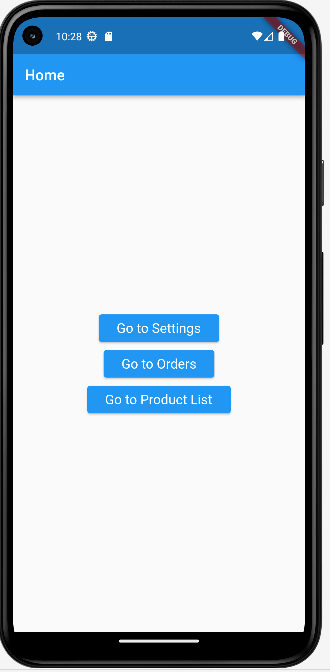
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( theme: ThemeData( elevatedButtonTheme: ElevatedButtonThemeData( style: ElevatedButton.styleFrom( padding: const EdgeInsets.symmetric( horizontal: 24, vertical: 8), // Adjust padding for button size textStyle: const TextStyle(fontSize: 18), // Adjust font size ), ), ), home: const HomeScreen(), ); } } class HomeScreen extends StatelessWidget { const HomeScreen({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text("Home"), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ ElevatedButton( onPressed: () { // Navigation Route home -> Route settings // Navigator.typeofNavigation(current location, destination); Navigator.push( context, MaterialPageRoute( builder: (context) => const SettingsScreen(), ), ); }, child: const Text("Go to Settings"), ), ElevatedButton( onPressed: () { Navigator.push( context, MaterialPageRoute( builder: (context) => const OrdersScreen(), ), ); }, child: const Text("Go to Orders"), ), ElevatedButton( onPressed: () { Navigator.push( context, MaterialPageRoute( builder: (context) => const ProductListScreen(), ), ); }, child: const Text("Go to Product List"), ), ], ), ), ); } } //Route settings class SettingsScreen extends StatelessWidget { const SettingsScreen({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text("Settings"), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ const Text( "Settings", style: TextStyle(fontSize: 24), ), ElevatedButton( onPressed: () { Navigator.pushAndRemoveUntil( context, MaterialPageRoute( builder: (context) => const HomeScreen()), (route) => false); }, child: const Text("Home")) ], ), ), ); } } // Route Orders class OrdersScreen extends StatelessWidget { const OrdersScreen({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text("Orders"), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ const Text( "Orders", style: TextStyle(fontSize: 24), ), TextButton( onPressed: () { Navigator.pushReplacement( context, MaterialPageRoute( builder: (context) => const SettingsScreen())); }, child: const Text(" Go to settings"), ), TextButton( onPressed: () { //Navigator.push(context, MaterialPageRoute(builder: (context)=>HomeScreen() )); Navigator.pop(context); }, child: const Text(" Go to Home"), ) ], ), ), ); } } // Route Product list class ProductListScreen extends StatelessWidget { const ProductListScreen({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text("Product list"), ), body: ListView.builder( itemCount: 20, itemBuilder: (context, index) { return ListTile( onTap: () { Navigator.push(context, MaterialPageRoute(builder: (context) { return ProductDetailsScreen(productName: index.toString()); // ignore: avoid_print })).then((value) => {print(value)}); }, title: Text(index.toString()), subtitle: Text("Product details $index"), ); }), ); } } // Route Product details class ProductDetailsScreen extends StatelessWidget { final String productName; const ProductDetailsScreen({Key? key, required this.productName}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text("Product Details"), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text( productName, style: const TextStyle(fontSize: 30), ), ElevatedButton( onPressed: () { Navigator.pop(context, "Product Name $productName"); //Navigator.push(context, MaterialPageRoute(builder: (context){ // return ProductListScreen(); // })); }, child: const Text("Bank to Product List")) ], ))); } }
Expanded, MediaQuery, Fittedbox widget and device_preview package.
Here we have to install device_preview from https://pub.dev/packages/device_preview
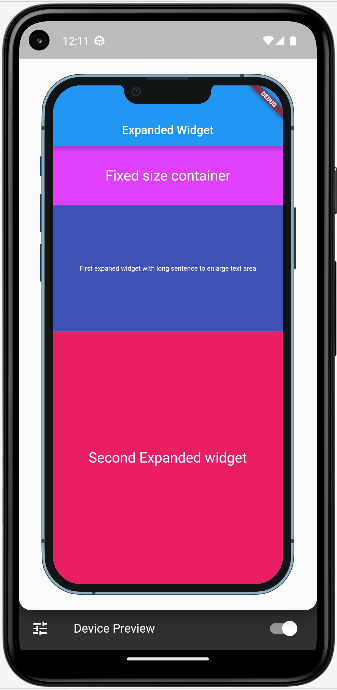
import 'dart:ui'; import 'package:flutter/material.dart'; import 'package:device_preview/device_preview.dart'; void main() { runApp( DevicePreview( enabled: true, builder: (context) => const MyApp(), ), ); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( home: HomeScreen(), ); } } class HomeScreen extends StatelessWidget { const HomeScreen({Key? key}) : super(key: key); //MediaQuery @override Widget build(BuildContext context) { // Size size = MediaQuery.of(context).size; Size size = MediaQuery.sizeOf(context); print(size.height); print(size.width); print(size.aspectRatio); print(size.flipped); Orientation orientation = MediaQuery.orientationOf(context); print(orientation); print(MediaQuery.devicePixelRatioOf(context)); return Scaffold( appBar: AppBar( title: Text("Expanded Widget"), centerTitle: true, ), body: Column( children: [ Container( alignment: Alignment.center, //width: MediaQuery.sizeOf(context).width, width: double.infinity, height: 100, color: Colors.purpleAccent, child: Text( "Fixed size container", style: TextStyle(color: Colors.white, fontSize: 24), ), ), Expanded( flex: 1, child: Container( width: MediaQuery.sizeOf(context).width, height: MediaQuery.sizeOf(context).width, child: Center( child: SizedBox( width: 300, height: 200, // Fittedbox is used to keep the text in given space! child: FittedBox( child: Text( 'First expaned widget with long sentence to enlarge text area', style: TextStyle(color: Colors.white, fontSize: 24), ), ), ), ), color: Colors.indigo, ), ), Expanded( flex: 2, child: Container( width: MediaQuery.sizeOf(context).width, height: MediaQuery.sizeOf(context).width, child: Center( child: Text( 'Second Expanded widget', style: TextStyle(color: Colors.white, fontSize: 24), ), ), color: Colors.pink, ), ), ], ), ); } } //MediaQuery @override Widget build(BuildContext context) { // Size size = MediaQuery.of(context).size; Size size = MediaQuery.sizeOf(context); print(size.height); print(size.width); print(size.aspectRatio); print(size.flipped); Orientation orientation = MediaQuery.orientationOf(context); print(orientation); print(MediaQuery.devicePixelRatioOf(context)); return Scaffold( appBar: AppBar( title: Text("Expanded Widget"), centerTitle: true, ), body: Column( children: [ Container( alignment: Alignment.center, //width: MediaQuery.sizeOf(context).width, width: double.infinity, height: 100, color: Colors.purpleAccent, child: Text("Fixed size container",style: TextStyle( color: Colors.white,fontSize: 24 ),), ), Expanded( flex: 1, child: Container( width: MediaQuery.sizeOf(context).width, height: MediaQuery.sizeOf(context).width, child: Center( child: SizedBox( width: 300, height: 200, // Fittedbox is used to keep the text in given space! child: FittedBox( child: Text( 'First expaned widget with long sentence to enlarge text area', style: TextStyle( color: Colors.white,fontSize: 24 ), ), ), ), ), color: Colors.indigo, ), ), Expanded( flex: 2, child: Container( width: MediaQuery.sizeOf(context).width, height: MediaQuery.sizeOf(context).width, child: Center( child: Text( 'Second Expanded widget', style: TextStyle( color: Colors.white,fontSize: 24 ), ), ), color: Colors.pink, ), ), ], ), ); }
Layout Builder in Flutter
The LayoutBuilder
widget in Flutter is used to get information about the constraints that are applied to a widget during the layout phase. It’s especially useful when you want to create custom layouts or widgets that need to adapt their size, position, or behavior based on the available space or constraints.
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( home: MyHome(), ); } } class MyHome extends StatelessWidget { const MyHome({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Home"), ), body: LayoutBuilder( builder: (BuildContext context, BoxConstraints constraints) { if (constraints.maxWidth > 600) { return Container( height: 400, width: 400, color: Colors.green, ); } else { return Container( height: 200, width: 200, color: Colors.red, ); } }, ), ); } }
FractionallySizedBox in Flutter
In Flutter, FractionallySizedBox
is a widget that sizes its child to a fraction of the total available space along both the horizontal and vertical axes. This can be useful when you want to create responsive layouts where the size of a child widget is relative to the available space
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( home: MyHome(), ); } } class MyHome extends StatelessWidget { const MyHome({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("FractionallySizedBox Testing"), ), body: Center( child: FractionallySizedBox( widthFactor: 0.5, heightFactor: 0.3, child: Container( color: Colors.green, ), ), )); } }
Simple Calculator using field controller and stateful widget
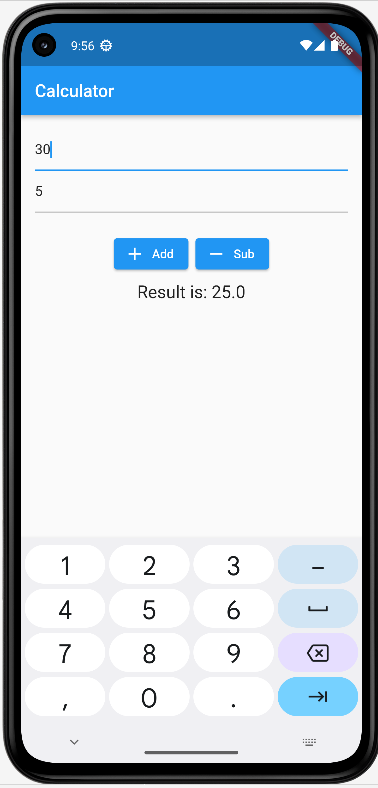
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( home: Home_Screen(), ); } } class Home_Screen extends StatefulWidget { const Home_Screen({Key? key}) : super(key: key); @override State<Home_Screen> createState() => _Home_ScreenState(); } class _Home_ScreenState extends State<Home_Screen> { TextEditingController _field1TEController = TextEditingController(); TextEditingController _field2TEController = TextEditingController(); GlobalKey<FormState> _formkey = GlobalKey<FormState>(); double result = 0; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Calculator"), ), body: Padding( padding: const EdgeInsets.all(16.0), child: Form( key: _formkey, child: Column( children: [ TextFormField( controller: _field1TEController, keyboardType: TextInputType.number, textInputAction: TextInputAction.next, decoration: const InputDecoration(hintText: 'Field 1'), validator: (String? value) { if (value?.isEmpty ?? true) { return 'Enter a valid value'; } return null; }, ), TextFormField( controller: _field2TEController, keyboardType: TextInputType.number, decoration: const InputDecoration(hintText: 'Field 1'), validator: (String? value) { if (value?.isEmpty ?? true) { return 'Enter a valid value'; } return null; }, ), const SizedBox( height: 16, ), ButtonBar( alignment: MainAxisAlignment.center, children: [ ElevatedButton.icon( onPressed: () { if (_formkey.currentState!.validate()) { double firstNumber = double.parse(_field1TEController.text.trim()); double lastNumber = double.parse(_field2TEController.text.trim()); result = add(firstNumber, lastNumber); setState(() {}); } }, icon: Icon(Icons.add), label: Text('Add'), ), ElevatedButton.icon( onPressed: () { if (_formkey.currentState!.validate()) { double firstNumber = double.parse(_field1TEController.text.trim()); double lastNumber = double.parse(_field2TEController.text.trim()); result = subtract(firstNumber, lastNumber); setState(() {}); } }, icon: Icon(Icons.remove), label: Text('Sub'), ), ], ), Text( 'Result is: $result', style: const TextStyle(fontSize: 20), ) ], ), ), ), ); } double add(double firstNumber, double lastNumber) { return firstNumber + lastNumber; } double subtract(double firstNumber, double lastNumber) { return firstNumber - lastNumber; } }
BottomNavigationBar
In this tutorial I have used the below screen/pages
- main.dart
- base.dart
- “screens” directory
- home_page.dart
- search_page.dart
- profile_page.dart
Now see the blow code to complete the project
main.dart
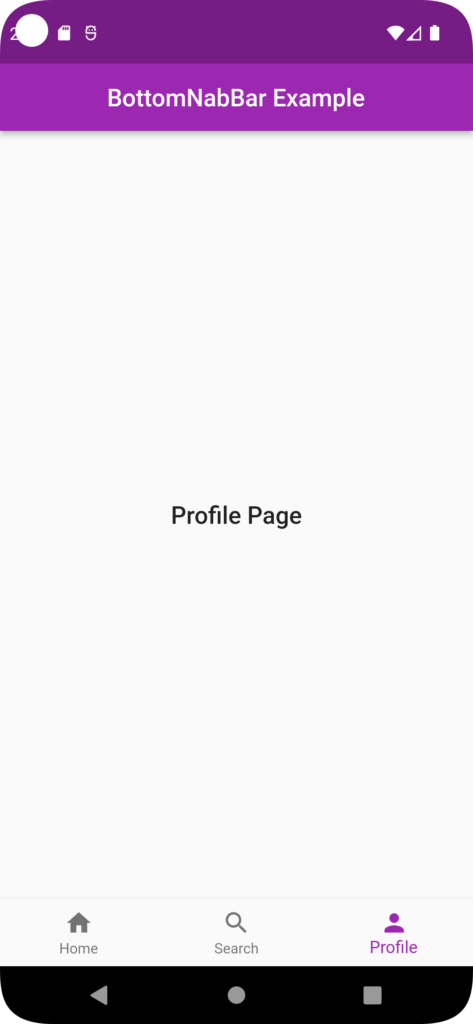
import 'package:flutter/material.dart'; import 'base.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, home: MyHomePage(), theme: ThemeData( primarySwatch: Colors.purple, ), ); } }
base.dart
import 'package:flutter/material.dart'; import 'package:tutor/screens/home_page.dart'; import 'package:tutor/screens/profile_page.dart'; import 'package:tutor/screens/search_page.dart'; class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { int _selectedIndex = 0; static const List<Widget> _widgetOptions = <Widget>[ HomePage(), SearchPage(), ProfilePage(), ]; void _onItemTapped(int index) { setState(() { _selectedIndex = index; }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( centerTitle: true, title: Text('BottomNabBar Example'), ), body: Center( child: _widgetOptions.elementAt(_selectedIndex), ), bottomNavigationBar: BottomNavigationBar( items: const <BottomNavigationBarItem>[ BottomNavigationBarItem( icon: Icon(Icons.home), label: 'Home', ), BottomNavigationBarItem( icon: Icon(Icons.search), label: 'Search', ), BottomNavigationBarItem( icon: Icon(Icons.person), label: 'Profile', ), ], currentIndex: _selectedIndex, selectedItemColor: Colors.purple, onTap: _onItemTapped, ), ); } }
home_page.dart
import 'package:flutter/material.dart'; class HomePage extends StatelessWidget { const HomePage({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Text("Home Page", style: Theme.of(context).textTheme.titleLarge), ), ); } }
search_page.dart
import 'package:flutter/material.dart'; class SearchPage extends StatelessWidget { const SearchPage({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Text('Search page', style: Theme.of(context).textTheme.titleLarge),), ); } }
profile_page.dart
import 'package:flutter/material.dart'; class ProfilePage extends StatelessWidget { const ProfilePage({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Text('Profile Page', style: Theme.of(context).textTheme.titleLarge),), ); } }