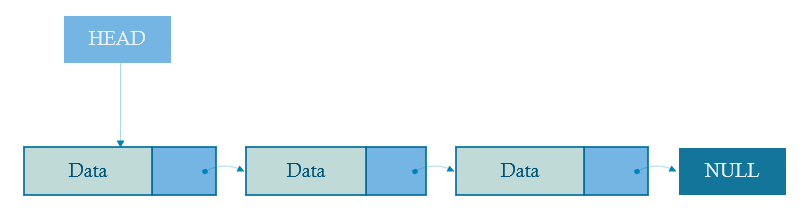
- What is a Linked List?
- A linked list is a data structure used to store a collection of elements.
- It consists of individual elements, called nodes, which are linked together in a linear sequence.
- Node in a Linked List:
- Each node in a linked list contains two parts:
- Data: The actual value or information you want to store.
- Pointer (or Reference): A reference to the next node in the sequence.
- Each node in a linked list contains two parts:
- Types of Linked Lists:
- There are different types of linked lists, including:
- Singly Linked List: Each node points to the next node.
- Doubly Linked List: Each node points to both the next and the previous node.
- Circular Linked List: The last node points back to the first node, forming a closed loop.
- There are different types of linked lists, including:
- Advantages of Linked Lists:
- Linked lists are dynamic data structures, which means they can grow or shrink as needed.
- They allow for efficient insertions and deletions at any position in the list.
- Drawbacks of Linked Lists:
- Accessing elements in a linked list may be slower than arrays since you have to traverse the list from the beginning.
- Linked lists use more memory than arrays because of the additional pointers.
- Example:
- Consider a singly linked list containing values: 10 -> 20 -> 30.
- In this list, the node with a value of 10 points to the node with a value of 20, and the node with a value of 20 points to the node with a value of 30. The last node points to
null
to indicate the end of the list.
- Operations on Linked Lists:
- Common operations include adding a node to the beginning or end, deleting a node, searching for a node with a specific value, and traversing the list to perform actions on each node.
- Use Cases:
- Linked lists are often used in situations where you need dynamic and efficient data storage, like in implementing data structures such as stacks, queues, and hash tables.
In summary, a linked list is a flexible data structure consisting of nodes connected in a sequence, making it useful for various applications where elements need to be stored and manipulated efficiently.
The following linked list example is written using Dart Programming language
import 'dart:collection'; base class Data<T> extends LinkedListEntry<Data<T>> { T value; Data(this.value); @override String toString() { return '$value'; } } void main() { // Create a list var linkedList = LinkedList<Data<int>>(); // Add elements to the end of the list linkedList.add(Data(20)); linkedList.add(Data(30)); linkedList.addFirst(Data(10)); // to add data in first position // Print the entire linked list print("linkedList is: $linkedList"); // Access a specific node (e.g., the second node at index 1) var currentNode = linkedList.elementAt(0); print("Current Node Value: ${currentNode.value}"); // Access the value of the next node var nextNode = currentNode.next; if (nextNode != null) { print("Next Node Value: ${nextNode.value}"); } else { print("No next node available."); } }