Table of Contents
Introduction
- jQuery is a JavaScript Library.
- jQuery simplifies huge complicated things from JavaScript, like AJAX calls and DOM manipulation.
- jQuery can be used by downloading the library or using CDN
- jQuery plugin is great helper to reduce your code and save time! link: https://plugins.jquery.com/
Prerequisites
You have to learn html, css and JavaScript at first before starting jQuery.
jQuery Syntax
jQuery Basic syntax is: $(selector).action()
Here selector is used to select HTML element and action() is an event to perform action on selected html elements.
Example: $(".data").hide()
– hides all elements with class=”data”.
A basic example is given below with explanation for better understanding.
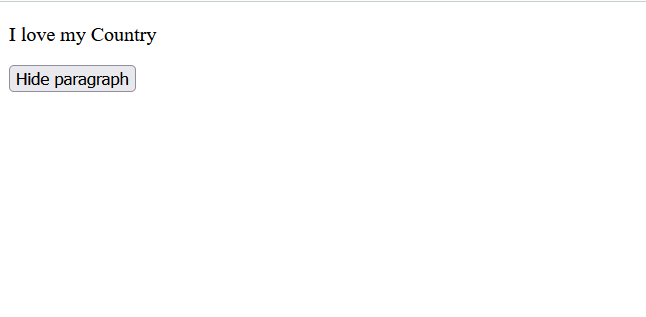
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script> <script> $(document).ready(function(){ $("button").click(function(){ $("p").hide(); }); }); </script> </head> <body> <p>I love my Country</p> <button>Hide paragraph</button> </body> </html>
Here jQuery library is included from the Google CDN (Content Delivery Network). It’s essential to include jQuery before using it in your JavaScript code.
$(document).ready() function ensures that the code runs when the document is fully loaded and ready.$(“button”).click(function(){ … });: This line is used to select all elements on the page and attaches a click event handler to them. $(“p”).hide();: This line selects all elements on the page and hides them when the button is clicked. The .hide() function is a jQuery method that sets the CSS display property of the selected elements to “none,” effectively hiding them from view.
So, when you load this HTML page in a web browser and click the “Hide paragraph” button, the element containing “I love my Country” will disappear from the page because it’s hidden using jQuery’s .hide() method.