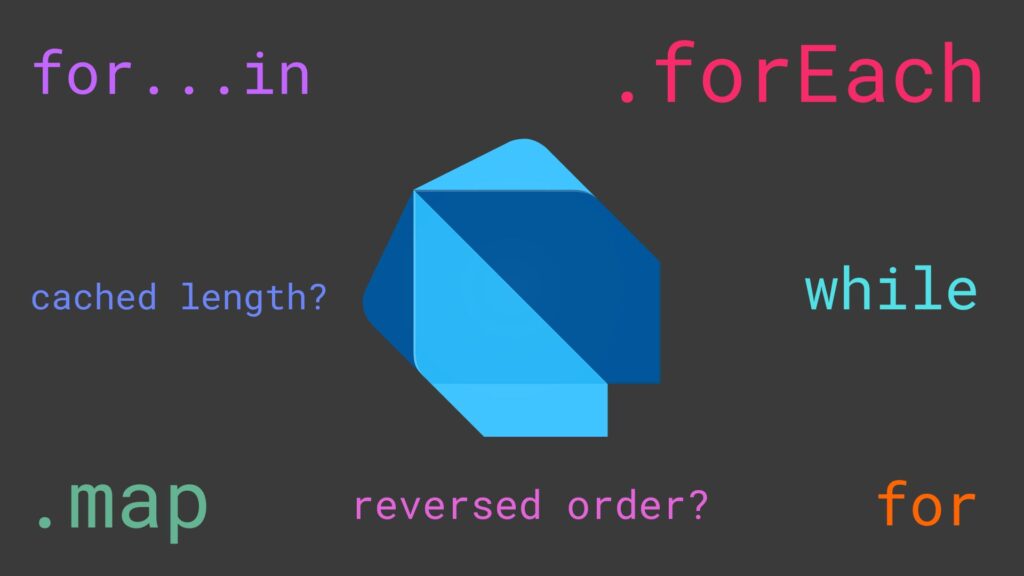
Table of Contents
Multiple input, List of Maps data structure
import 'dart:io'; void main() { List<Map<String, dynamic>> priceLists = [ {'product_id': '101', 'price': 10}, {'product_id': '202', 'price': 25}, {'product_id': '303', 'price': 5} ]; String? inputLine = stdin.readLineSync(); List<String> data = inputLine!.split(' '); String I = data[0]; int Q = int.parse(data[1]); if (Q >= 0 && Q <= 20000) { for (var priceList in priceLists) { if (priceList['product_id'] == I) { int result = priceList['price'] * Q; print(result); break; } } } else { print("Out of Range"); } }
Map Data Type [Problem: Identify Fruit name according to Fruit ID]
import 'dart:io'; void main() { Map<int, String> fruitMap = { 31231: "Banana", 23456: "Apple", 78901: "Mango", 45678: "Orange", }; int fruitId = int.parse(stdin.readLineSync()!); if (fruitMap.containsKey(fruitId)) { String fruitName = fruitMap[fruitId]!; print(fruitName); } else { print("Fruit not found"); } }
Problem Statement
Write a program to create a function that takes two arguments: the original price and the discount percentage as integers and returns the final price after the discount.
The input consists of two integers: the original price and the discount.
The output will print the final price after discount which will be a float number.
import 'dart:io'; double calculateFinalPrice(int originalPrice, int discount) { if (originalPrice < 0) { return 0.0; // Ensure the price is non-negative } if (discount < 0 || discount > 100) { return originalPrice.toDouble(); // No discount or invalid discount percentage } double discountAmount = originalPrice * (discount / 100); double finalPrice = originalPrice - discountAmount; return finalPrice; } void main() { // Read multiple input in same line String input = stdin.readLineSync()!; List<int> parts = input.split(' ').map(int.parse).toList(); int originalPrice = parts[0]; int discount = parts[1]; double finalPrice = calculateFinalPrice(originalPrice, discount); print('Price: ${finalPrice.toStringAsFixed(2)}'); }